Node.js で Azure Cosmos DBに接続する
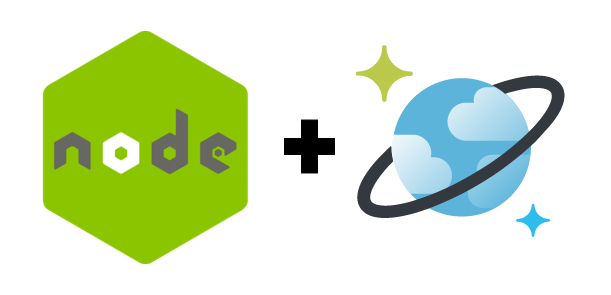
Node.js の勉強で簡単なwebアプリを作成した。その際にローカル環境ではmongoDBを使用していたんだけど、Azureにデプロイして使用する際にはAzure Cosmos DBを使用しようと思ったのでやり方をメモしようと思います。
Azure Cosmos DB を作成する
Azure Cosmos DB アカウントの作成をクリックします。

- API:MongoDB用 Azure Cosmos DB API
- 場所:(アジア太平洋)東日本
確認と作成をクリックします。

作成完了後、コレクションの参照をクリックします。

New Collection をクリックします。
- Database id: DB名
- Collection id: コレクション名
- Storage capacity: Fixed(10GB) ※制限なく使用する場合はUnlimited
入力後、OKをクリックします。

Azure Cosmos DB に接続する
クイックスタートをクリックして、Node.js → プライマリ接続文字列の順でクリックします。

プライマリ接続文字列をコピーします。コピー後にポート番号の後ろに先ほど作成したDB名を入れます。
・・・documents.azure.com:10255/{先ほど作成したDB名}・・・
以下のスクリプトを実行します。
var CONNECTION_URL = 接続文字列;
var DATABASE = "先ほど作成したDB名";
var OPTIONS = {
family: 4,
useNewUrlParser:"true"
};
var MongoClient = require("mongodb").MongoClient;
// posts,users,privileges
var insertPosts = function (db) {
return Promise.all([
db.collection("posts").insertMany([
{
url: "/2019/05/01/hello-node.js.html",
published: new Date(2019, 5, 1),
updated: new Date(2019, 5, 1),
title: "2019年5月1日の日記",
content: "2019年5月1日の日記",
keywords: ["Node.js"],
authors: ["TARO TANAKA"]
},
{
url: "/2019/05/02/hello-node.js.html",
published: new Date(2019, 5, 2),
updated: new Date(2019, 5, 2),
title: "2019年5月2日の日記",
content: "2019年5月2日の日記",
keywords: ["Node.js"],
authors: ["TARO ITO"]
},
{
url: "/2019/05/03/hello-node.js.html",
published: new Date(2019, 5, 3),
updated: new Date(2019, 5, 3),
title: "2019年5月3日の日記",
content: "2019年5月3日の日記",
keywords: ["Node.js"],
authors: ["TARO SAITO"]
}
]),
db.collection("posts").createIndex({ url: 1 }, { unique: true, background: true })
]);
};
var insertUsers = function (db) {
return Promise.all([
db.collection("users").insertMany([
{
email:"sample@sample.com",
name:"YUTAKA SATO",
password:"345489c72fda6ec55971274b62922442e5af13980503b052af735f6cf805a9e3",//AAAAA,
role:"owner"
}
]),
db.collection("users").createIndex({ email: 1 }, { unique: true, background: true })
]);
};
var insertPrivileges = function (db) {
return Promise.all([
db.collection("privileges").insertMany([
{role:"default",permissions:["read"]},
{role:"owner",permissions:["readWrite"]}
]),
db.collection("privileges").createIndex({ role: 1 }, { unique: true, background: true })
]);
};
MongoClient.connect(CONNECTION_URL, OPTIONS, (error, client) => {
var db = client.db(DATABASE);
Promise.all([
insertPosts(db),
insertUsers(db),
insertPrivileges(db)
]).catch((error) => {
console.log(error);
}).then(() => {
client.close();
});
});
適当に値を取得してみます。
var CONNECTION_URL = 接続文字列;
var DATABASE = "先ほど作成したDB名";
var OPTIONS = {
family: 4,
useNewUrlParser: "true"
};
var MongoClient = require("mongodb").MongoClient;
MongoClient.connect(CONNECTION_URL, OPTIONS, (error, client) => {
var db = client.db(DATABASE);
db.collection("posts")
.find().toArray((error, documents) => {
for (var document of documents) {
console.log(document.url);
}
});
db.collection("users")
.find().toArray((error, documents) => {
for (var document of documents) {
console.log(document.email);
}
});
db.collection("privileges")
.find().toArray((error, documents) => {
for (var document of documents) {
console.log(document.role);
}
});
});
先ほどのデータが正常に入っていることがわかります。

以上で Node.js で Azure Cosmos DBに接続するは終了になります。Node.js の勉強で簡単なWebアプリを作成したので、次回はAzureにWebアプリをデプロイする作業を説明していこうと思います。